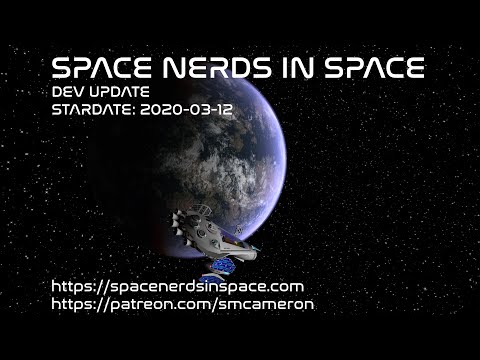
smcameron {l Wrote}:Not sure it's a great idea to invite people at a conference to touch your keyboards with this corona virus thing out there.
static void add_sp_entry(struct space_partition *p, struct space_partition_entry *e, int cell)
{
struct space_partition_entry **c;
if (cell < 0)
c = &p->common; <--- notice the ampersand here. c is the address of common.
else
c = &p->content[cell];
e->cell = cell;
if (!(*c)) {
(*c) = e; <--- common gets initialized here.
e->next = NULL;
e->prev = NULL;
return;
}
(*c)->prev = e;
e->next = *c;
e->prev = NULL;
(*c) = e;
}
p = &go[i];
if (p->type != OBJTYPE_PLANET) {
/* This can happen if a planet gets destroyed and another object re-uses the ID. */
o->tsd.starbase.associated_planet_id = -1;
return;
}
smcameron {l Wrote}:Another quick update. Added a warp gate effect, not a big deal but better than the nothing that was there before, and also now NPC ships are respawned at warp gates instead of at just random locations.
https://youtu.be/t1Vhyg9SCjU
if (nsparks > 40) { /* a big explosion, add one big freakin' stationary spark that fades quickly */
add_spark(x, y, z, 0, 0, 0, 15, color, &spark_material, 0.8, 0, 250.0);
particle_mesh = mesh_fabricate_billboard(50.0f, 50.0f);
/* For each boundary candidate, count large object intersections */
if (pass == 0 && n_passes > 1) {
if (e->m->radius * max_scale < 500)
continue; /* ignore small objects in choosing render pass boundary */
camera_to_entity.v.x = e->x - camera_pos.v.x;
camera_to_entity.v.y = e->y - camera_pos.v.y;
camera_to_entity.v.z = e->z - camera_pos.v.z;
float zdist = vec3_dot(&camera_to_entity, &camera_look);
if (zdist < 0)
continue; /* behind camera, ignore (should already be frustum culled). */
if (e->m->geometry_mode == MESH_GEOMETRY_POINTS) /* Ignore fake stars, etc. */
continue;
if (e->m->radius * max_scale >= 299999.0)
continue; /* Hack to ignore warp tunnels (r=300000) */
/* Check each candidate boundary for intersection with this object */
for (k = 0; k < 3; k++)
if (fabsf(zdist - boundary_candidate[k]) < e->m->radius * max_scale)
boundary_intersects[k]++;
}
}
if (pass == 0 && n_passes > 1) {
/* Find the boundary candidate with the fewest large object intersections */
last_candidate = candidate;
candidate = 0;
if (boundary_intersects[candidate] != 0) {
/* Test for less than or *equal* so we choose the *farthest* among equals so
* that any resulting artifacts are more likely to occupy less screen space
* and be less obnoxious. With a little luck, it will even move it to a place
* that is invisible, like the back side of a planet.
*/
if (boundary_intersects[1] <= boundary_intersects[candidate])
candidate = 1;
if (boundary_intersects[2] <= boundary_intersects[candidate])
candidate = 2;
}
if (last_candidate != candidate) {
/* Recalculate the camera transforms if the render pass boundary was changed
to avoid some intersecting object */
render_pass_boundary = boundary_candidate[candidate];
calculate_camera_transform_near_far(&cx->camera, &rendering_pass[0],
render_pass_boundary, cx->camera.far);
calculate_camera_transform_near_far(&cx->camera, &rendering_pass[1],
cx->camera.near, render_pass_boundary + render_pass_boundary / 1000.0);
}
}
Users browsing this forum: No registered users and 1 guest